In this section, we’ll show you how to build a credit card payment form using Bootstrap 5. Additionally, we’ll design a user-friendly Bootstrap 5 form for entering credit card details, featuring icons and a floating label for each input field.
Starting Bootstrap 5 Structure with CDN
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<title>Bootstrap 5 Credit Card Form Example</title>
<link
href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity="sha384-T3c6CoIi6uLrA9TneNEoa7RxnatzjcDSCmG1MXxSR1GAsXEV/Dwwykc2MPK8M2HN"
crossorigin="anonymous"
/>
</head>
<body>
<!-- content -->
<script
src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/js/bootstrap.bundle.min.js"
integrity="sha384-C6RzsynM9kWDrMNeT87bh95OGNyZPhcTNXj1NW7RuBCsyN/o0jlpcV8Qyq46cDfL"
crossorigin="anonymous"
></script>
</body>
</html>
Credit Card Payment Form Using Plan Bootstrap 5
HTML
<div class="container mt-5">
<div class="row">
<div class="col-md-6 offset-md-3">
<div class="card">
<div class="card-body">
<h5 class="card-title">Credit Card Payment</h5>
<form>
<div class="mb-3">
<label for="cardNumber" class="form-label">Card Number</label>
<input type="text" class="form-control" id="cardNumber" placeholder="0000 0000 0000 0000" />
</div>
<div class="mb-3">
<label for="cardName" class="form-label">Name on Card</label>
<input type="text" class="form-control" id="cardName" placeholder="John Doe" />
</div>
<div class="row">
<div class="col-md-6 mb-3">
<label for="expiryMonth" class="form-label">Expiry Month</label>
<input type="text" class="form-control" id="expiryMonth" placeholder="MM" />
</div>
<div class="col-md-6 mb-3">
<label for="expiryYear" class="form-label">Expiry Year</label>
<input type="text" class="form-control" id="expiryYear" placeholder="YYYY" />
</div>
</div>
<div class="mb-3">
<label for="cvv" class="form-label">CVV</label>
<input type="text" class="form-control" id="cvv" placeholder="123" />
</div>
<button type="submit" class="btn btn-primary">
Submit Payment
</button>
</form>
</div>
</div>
</div>
</div>
</div>
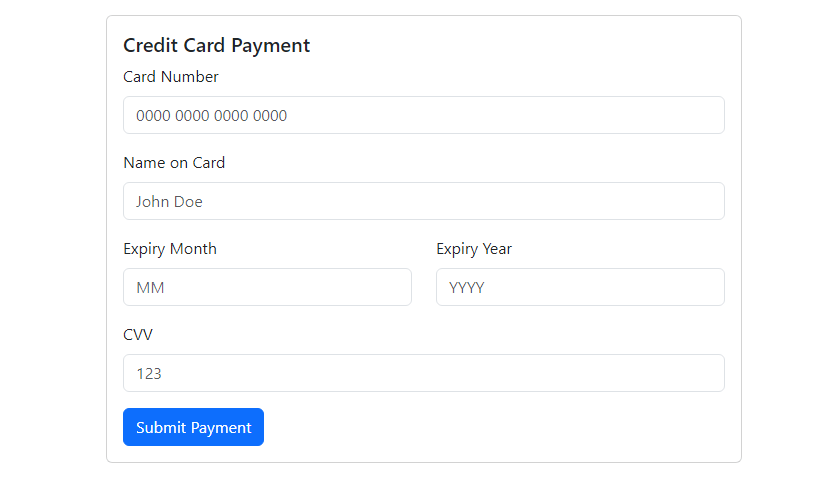
Add Bootstrap 5 Icon CDN
HTML
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap-icons@1.11.1/font/bootstrap-icons.css">
Bootstrap 5 Credit Card Form with Inline Inputs and Icons
HTML
<div class="container mt-5">
<div class="card">
<div class="card-body">
<h5 class="card-title">Credit Card Information</h5>
<form>
<div class="mb-3">
<label for="cardNumber" class="form-label">Card Number</label>
<div class="input-group">
<span class="input-group-text"><i class="bi bi-credit-card"></i></span>
<input type="text" class="form-control" id="cardNumber" placeholder="0000 0000 0000 0000">
</div>
</div>
<div class="mb-3">
<label for="cardName" class="form-label">Name on Card</label>
<input type="text" class="form-control" id="cardName" placeholder="John Doe">
</div>
<div class="row">
<div class="col-md-4 mb-3">
<label for="expiryMonth" class="form-label">Expiry Month</label>
<input type="text" class="form-control" id="expiryMonth" placeholder="MM">
</div>
<div class="col-md-4 mb-3">
<label for="expiryYear" class="form-label">Expiry Year</label>
<input type="text" class="form-control" id="expiryYear" placeholder="YYYY">
</div>
<div class="col-md-4 mb-3">
<label for="cvv" class="form-label">CVV</label>
<input type="text" class="form-control" id="cvv" placeholder="123">
</div>
</div>
<button type="submit" class="btn btn-success">Pay Now</button>
</form>
</div>
</div>
</div>
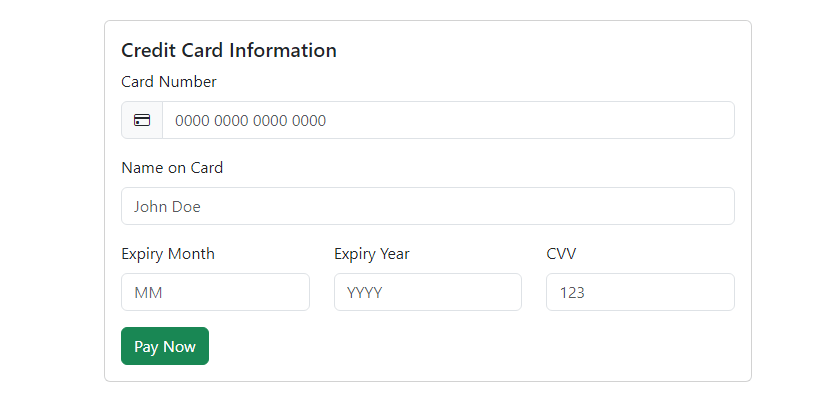
Bootstrap 5 Credit Card Form with Floating Labels
HTML
<div class="container mt-5">
<div class="card">
<div class="card-header">Secure Payment</div>
<div class="card-body">
<form>
<div class="form-floating mb-3">
<input type="text" class="form-control" id="floatingCardNumber" placeholder="Card Number" />
<label for="floatingCardNumber">Card Number</label>
</div>
<div class="form-floating mb-3">
<input type="text" class="form-control" id="floatingCardName" placeholder="Name on Card" />
<label for="floatingCardName">Name on Card</label>
</div>
<div class="row g-2">
<div class="col-md">
<div class="form-floating">
<input type="text" class="form-control" id="floatingExpiryMonth"
placeholder="Expiry Month" />
<label for="floatingExpiryMonth">Expiry Month</label>
</div>
</div>
<div class="col-md">
<div class="form-floating">
<input type="text" class="form-control" id="floatingExpiryYear" placeholder="Expiry Year" />
<label for="floatingExpiryYear">Expiry Year</label>
</div>
</div>
<div class="col-md">
<div class="form-floating">
<input type="text" class="form-control" id="floatingCvv" placeholder="CVV" />
<label for="floatingCvv">CVV</label>
</div>
</div>
</div>
<button type="submit" class="btn btn-primary mt-3">
Proceed to Pay
</button>
</form>
</div>
</div>
</div>
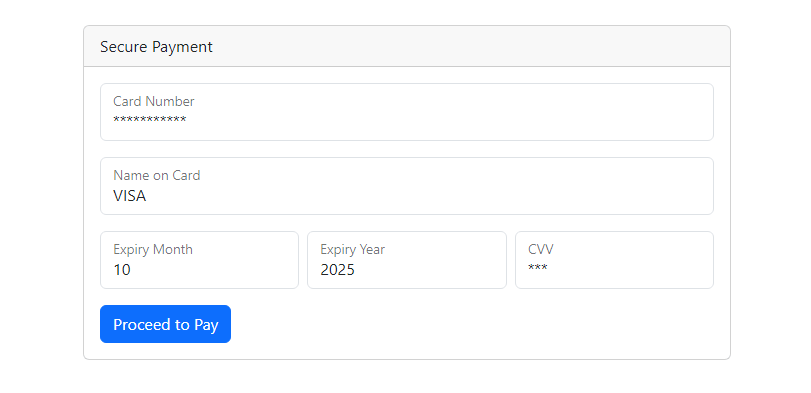
Javed sheikh
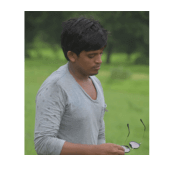
Hello there! I’m Javed Sheikh, a frontend developer with a passion for crafting seamless user experiences. With expertise in JavaScript frameworks like Vue.js, Svelte, and React, I bring creativity and innovation to every project I undertake. From building dynamic web applications to optimizing user interfaces,