In this tutorial, we’ll create search bar in Nuxt 3 using Tailwind CSS. Before getting started, make sure you’ve installed and configured Tailwind CSS in your Nuxt 3 project.
Install Tailwind CSS in Nuxt 3 with NuxtTailwind Module
NuxtJS with Tailwind CSS Search Bar
Vue
<template>
<div>
<input
v-model="query"
@input="search"
type="text"
placeholder="Search..."
class="px-4 py-2 border rounded-md"
/>
</div>
</template>
<script setup>
const query = ref('');
const search = () => {
// Implement your search logic here
console.log('Searching for:', query.value);
};
</script>
NuxtJS 3 with Typescript Search bar using Tailwind CSS.
Vue
<template>
<div>
<input
v-model="query"
@input="search"
type="text"
placeholder="Search..."
class="px-4 py-2 border rounded-md"
/>
</div>
</template>
<script setup lang="ts">
import { ref } from 'vue';
const query = ref<string>('');
const search = () => {
// Implement your search logic here
console.log('Searching for:', query.value);
};
</script>

Nuxt 3 TypeScript with Tailwind CSS Search Bar with Icon.
Vue
<template>
<div class="flex items-center">
<input
type="text"
placeholder="Search..."
v-model="searchQuery"
class="border p-2 rounded-l focus:outline-none focus:border-blue-500"
/>
<button @click="handleSearch" class="p-2 rounded border text-gray-400">
<svg
xmlns="http://www.w3.org/2000/svg"
fill="none"
viewBox="0 0 24 24"
stroke-width="1.5"
stroke="currentColor"
class="w-6 h-6"
>
<path
stroke-linecap="round"
stroke-linejoin="round"
d="M21 21l-5.197-5.197m0 0A7.5 7.5 0 105.196 5.196a7.5 7.5 0 0010.607 10.607z"
/>
</svg>
</button>
</div>
</template>
<script setup lang="ts">
const searchQuery = ref("");
const handleSearch = () => {
console.log("Search:", searchQuery.value);
// Implement your search logic here
};
</script>

Implementing a Search Bar with Inside Icon in a Nuxt 3 TypeScript Project Using Tailwind CSS.
Vue
<template>
<div class="flex items-center">
<div class="relative">
<input
type="text"
placeholder="Search..."
v-model="searchQuery"
class="border p-2 rounded-md focus:outline-none focus:border-blue-500 pr-8"
/>
<button @click="handleSearch" class="absolute inset-y-0 right-0 p-2 rounded text-gray-400">
<svg
xmlns="http://www.w3.org/2000/svg"
fill="none"
viewBox="0 0 24 24"
stroke-width="1.5"
stroke="currentColor"
class="w-6 h-6"
>
<path
stroke-linecap="round"
stroke-linejoin="round"
d="M21 21l-5.197-5.197m0 0A7.5 7.5 0 105.196 5.196a7.5 7.5 0 0010.607 10.607z"
/>
</svg>
</button>
</div>
</div>
</template>
<script setup lang="ts">
const searchQuery = ref("");
const handleSearch = () => {
console.log("Search:", searchQuery.value);
// Implement your search logic here
};
</script>

Nuxt 3 TypeScript with Tailwind CSS Search Bar and Fake API Integration.
Vue
<template>
<div class="flex items-center flex-col m-8">
<div class="relative">
<input
type="text"
placeholder="Search..."
v-model="searchQuery"
class="border p-2 rounded-md focus:outline-none focus:border-blue-500 pr-8"
/>
<button @click="handleSearch" class="absolute inset-y-0 right-0 p-2 rounded text-gray-400">
<svg
xmlns="http://www.w3.org/2000/svg"
fill="none"
viewBox="0 0 24 24"
stroke-width="1.5"
stroke="currentColor"
class="w-6 h-6"
>
<path
stroke-linecap="round"
stroke-linejoin="round"
d="M21 21l-5.197-5.197m0 0A7.5 7.5 0 105.196 5.196a7.5 7.5 0 0010.607 10.607z"
/>
</svg>
</button>
</div>
<!-- Show results section -->
<div v-if="results.length" class="mt-4">
<h2 class="text-xl font-semibold">Search Results:</h2>
<ul>
<li v-for="result in results" :key="result.id">
{{ (result as any).title }}
</li>
</ul>
</div>
</div>
</template>
<script setup lang="ts">
import { ref } from 'vue';
interface SearchResult {
id: number;
title: string;
// Add other properties as needed
}
const searchQuery = ref('');
const results = ref<SearchResult[]>([]);
const handleSearch = async () => {
try {
const response = await fetch(`https://jsonplaceholder.typicode.com/posts?q=${searchQuery.value}`);
const data = await response.json();
results.value = data;
} catch (error) {
console.error('Error during search:', error);
}
};
</script>
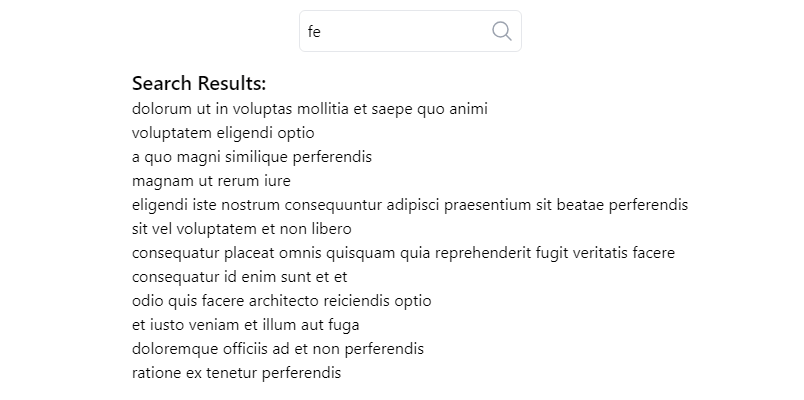