In this tutorial, we’ll creating an Add to Cart page using Bootstrap 5. We’ll cover various aspects, including shopping cart functionality, product listing in a table, checkout process, and implementing a product grid with an Add to Cart modal.
Starting Bootstrap 5 Structure with CDN
HTML
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Bootstrap 5 add to cart page</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-rbsA2VBKQhggwzxH7pPCaAqO46MgnOM80zW1RWuH61DGLwZJEdK2Kadq2F9CUG65" crossorigin="anonymous">
</head>
<body>
<h1>Hello, world!</h1>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js" integrity="sha384-kenU1KFdBIe4zVF0s0G1M5b4hcpxyD9F7jL+jjXkk+Q2h455rYXK/7HAuoJl+0I4" crossorigin="anonymous"></script>
</body>
</html>
Building a Basic and Responsive Add to Cart Page with Plain Bootstrap 5
HTML
<div class="container py-4">
<div class="row">
<div class="col-md-8">
<h2 class="mb-4">Our Products</h2>
<div class="row row-cols-1 row-cols-md-3 g-4">
<div class="col">
<div class="card h-100">
<img src="http://via.placeholder.com/640x360" class="card-img-top" alt="Product Image" />
<div class="card-body">
<h5 class="card-title">Product Name</h5>
<p class="card-text">
Some quick example text to build on the card title and make up
the bulk of the card's content.
</p>
<div class="d-flex justify-content-between align-items-center">
<div class="btn-group">
<button type="button" class="btn btn-sm btn-outline-secondary">
View
</button>
<button type="button" class="btn btn-sm btn-outline-secondary">
Add to Cart
</button>
</div>
<small class="text-muted">$19.99</small>
</div>
</div>
</div>
</div>
<div class="col">
<div class="card h-100">
<img src="http://via.placeholder.com/640x360" class="card-img-top" alt="Product Image" />
<div class="card-body">
<h5 class="card-title">Product Name</h5>
<p class="card-text">
Some quick example text to build on the card title and make up
the bulk of the card's content.
</p>
<div class="d-flex justify-content-between align-items-center">
<div class="btn-group">
<button type="button" class="btn btn-sm btn-outline-secondary">
View
</button>
<button type="button" class="btn btn-sm btn-outline-secondary">
Add to Cart
</button>
</div>
<small class="text-muted">$19.99</small>
</div>
</div>
</div>
</div>
<div class="col">
<div class="card h-100">
<img src="http://via.placeholder.com/640x360" class="card-img-top" alt="Product Image" />
<div class="card-body">
<h5 class="card-title">Product Name</h5>
<p class="card-text">
Some quick example text to build on the card title and make up
the bulk of the card's content.
</p>
<div class="d-flex justify-content-between align-items-center">
<div class="btn-group">
<button type="button" class="btn btn-sm btn-outline-secondary">
View
</button>
<button type="button" class="btn btn-sm btn-outline-secondary">
Add to Cart
</button>
</div>
<small class="text-muted">$19.99</small>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="col-md-4">
<div class="card">
<div class="card-header">Cart Summary</div>
<ul class="list-group list-group-flush">
<li class="list-group-item">
Product Name 1
<span class="badge bg-primary float-end">$19.99</span>
</li>
<li class="list-group-item">
Product Name 2
<span class="badge bg-primary float-end">$39.99</span>
</li>
<li class="list-group-item">
<strong>Total</strong>
<span class="badge bg-success float-end">$59.98</span>
</li>
</ul>
<div class="card-body">
<a href="#" class="btn btn-primary">Checkout</a>
</div>
</div>
</div>
</div>
</div>
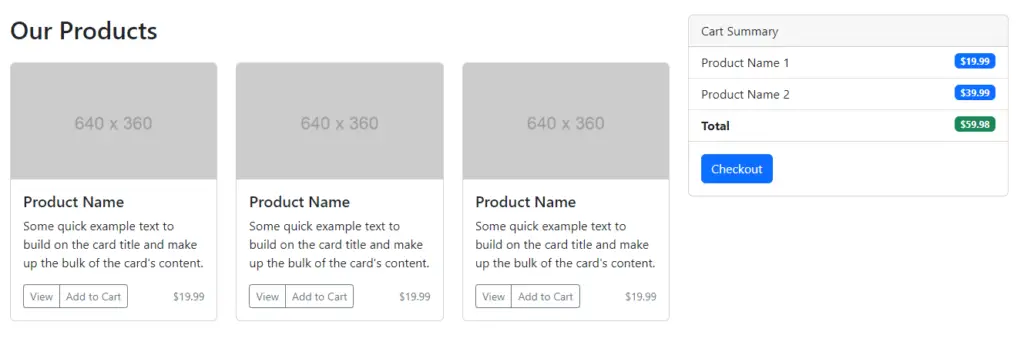
Product Grid with Add to Cart Modal
We’ll create a product grid where each product will have an Add to Cart button. Clicking this button will add the product to a cart which can be reviewed in a modal dialog.
HTML
<div class="container py-4">
<h2 class="mb-4">Our Products</h2>
<div class="row g-3">
<div class="col-md-4">
<div class="card">
<img src="http://via.placeholder.com/640x360" class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Product Title</h5>
<p class="card-text">Product description...</p>
<button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#cartModal">Add
to Cart</button>
</div>
</div>
</div>
<div class="col-md-4">
<div class="card">
<img src="http://via.placeholder.com/640x360" class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Product Title</h5>
<p class="card-text">Product description...</p>
<button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#cartModal">Add
to Cart</button>
</div>
</div>
</div>
<div class="col-md-4">
<div class="card">
<img src="http://via.placeholder.com/640x360" class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Product Title</h5>
<p class="card-text">Product description...</p>
<button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#cartModal">Add
to Cart</button>
</div>
</div>
</div>
</div>
</div>
<!-- Cart Modal -->
<div class="modal fade" id="cartModal" tabindex="-1" aria-labelledby="cartModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="cartModalLabel">Shopping Cart</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
Your cart is empty.
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-bs-dismiss="modal">Continue Shopping</button>
<button type="button" class="btn btn-primary">Checkout</button>
</div>
</div>
</div>
</div>
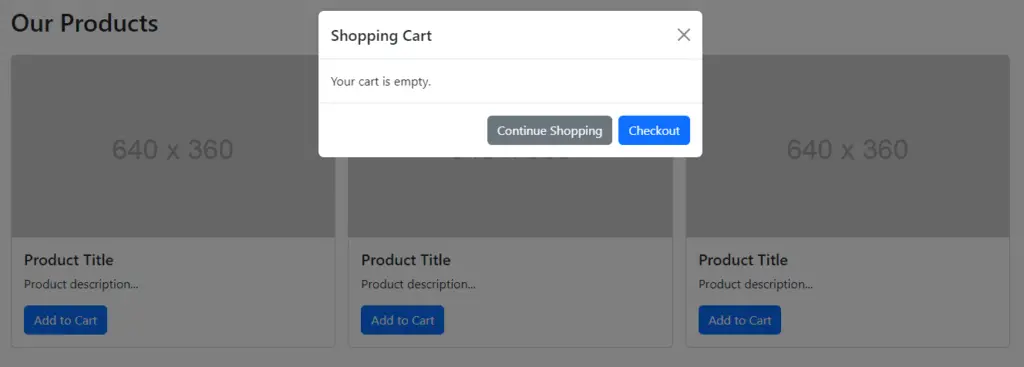
Product Table with Sidebar Cart
This example uses a table to list products and a sidebar to show the cart, suitable for a single-page application where the cart is always visible.
HTML
<div class="container py-4">
<div class="row">
<div class="col-md-8">
<h2 class="mb-4">Product List</h2>
<table class="table">
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Product</th>
<th scope="col">Price</th>
<th scope="col">Add</th>
</tr>
</thead>
<tbody>
<tr>
<th scope="row">1</th>
<td>Example Product</td>
<td>$9.99</td>
<td><button class="btn btn-primary">Add to Cart</button></td>
</tr>
<tr>
<th scope="row">1</th>
<td>Example Product</td>
<td>$9.99</td>
<td><button class="btn btn-primary">Add to Cart</button></td>
</tr>
<tr>
<th scope="row">1</th>
<td>Example Product</td>
<td>$9.99</td>
<td><button class="btn btn-primary">Add to Cart</button></td>
</tr>
</tbody>
</table>
</div>
<div class="col-md-4">
<h2 class="mb-4">Cart</h2>
<ul class="list-group mb-3">
<li class="list-group-item d-flex justify-content-between lh-sm">
<div>
<h6 class="my-0">Product name</h6>
<small class="text-muted">Brief description</small>
</div>
<span class="text-muted">$12</span>
</li>
<!-- Cart Items End -->
<li class="list-group-item d-flex justify-content-between">
<span>Total (USD)</span>
<strong>$20</strong>
</li>
</ul>
<button class="btn btn-primary btn-lg" type="submit">Checkout</button>
</div>
</div>
</div>
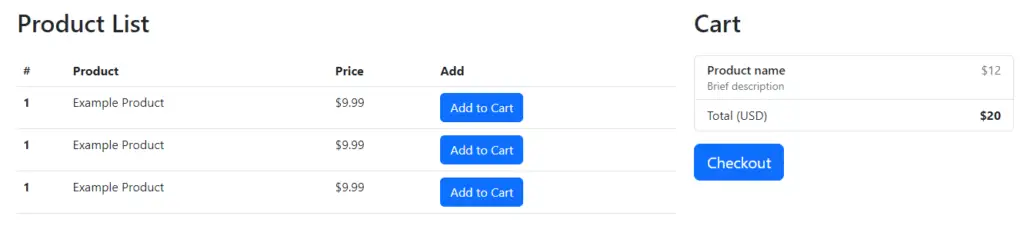