In this tutorial, we’ll create a login form with an image using Bootstrap 5. We’ll also see login forms with background images and side images for added visual appeal.
Starting Bootstrap 5 Structure with CDN
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Bootstrap 5 login Form with Image </title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-rbsA2VBKQhggwzxH7pPCaAqO46MgnOM80zW1RWuH61DGLwZJEdK2Kadq2F9CUG65" crossorigin="anonymous">
</head>
<body>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js" integrity="sha384-kenU1KFdBIe4zVF0s0G1M5b4hcpxyD9F7jL+jjXkk+Q2h455rYXK/7HAuoJl+0I4" crossorigin="anonymous"></script>
</body>
</html>
Create a Bootstrap 5 login form with image using pixabay image.
<div class="container">
<div class="row justify-content-center align-items-center" style="min-height: 100vh;">
<div class="col-6">
<div class="card">
<img src="https://cdn.pixabay.com/photo/2023/10/20/19/24/path-8330103_640.jpg" class="card-img-top" alt="image">
<div class="card-body">
<h5 class="card-title">Login</h5>
<form>
<div class="mb-3">
<label for="email" class="form-label">Email address</label>
<input type="email" class="form-control" id="email" aria-describedby="emailHelp">
</div>
<div class="mb-3">
<label for="password" class="form-label">Password</label>
<input type="password" class="form-control" id="password">
</div>
<div class="mb-3 form-check">
<input type="checkbox" class="form-check-input" id="rememberMe">
<label class="form-check-label" for="rememberMe">Remember me</label>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
</div>
</div>
</div>
</div>
</div>
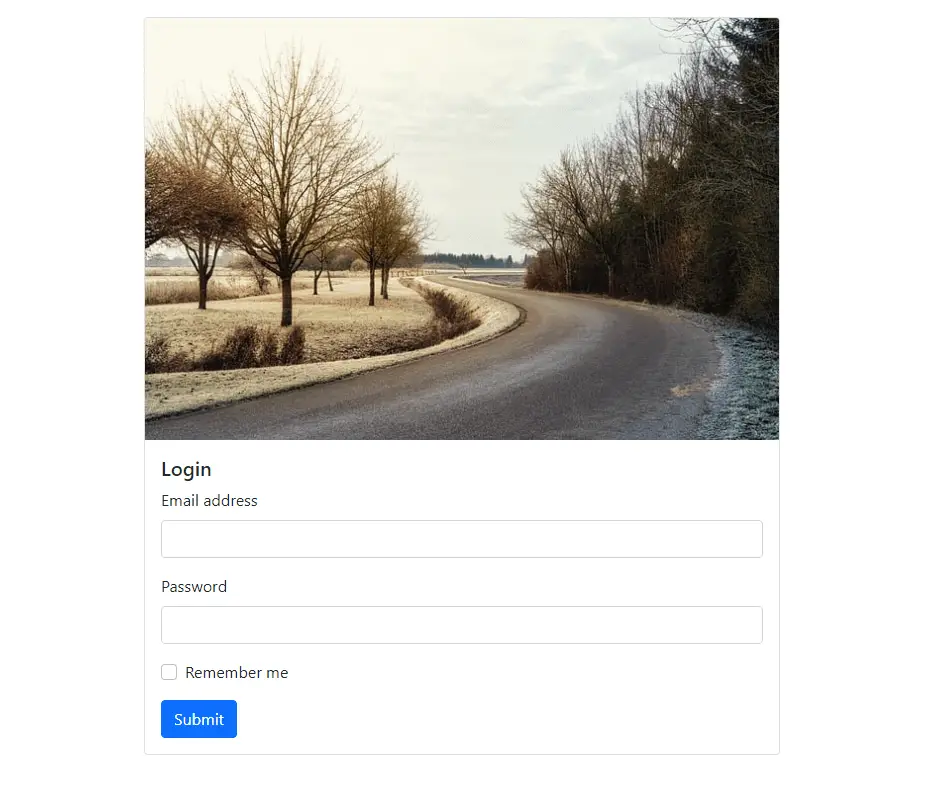
Bootstrap 5 Login Form with Full-Width Background Image
Create a Bootstrap 5 login form with a semi-transparent overlay on a full-width background image, along with some custom CSS to style it.
<div class="login-container">
<div class="login-form">
<h3 class="mb-4">Login to Your Account</h3>
<form>
<div class="mb-3">
<input type="email" class="form-control" placeholder="Email Address" required>
</div>
<div class="mb-3">
<input type="password" class="form-control" placeholder="Password" required>
</div>
<div class="mb-3 form-check">
<input type="checkbox" class="form-check-input" id="rememberMe">
<label class="form-check-label" for="rememberMe">Remember me</label>
</div>
<div class="d-grid">
<button type="submit" class="btn btn-primary">Sign In</button>
</div>
</form>
</div>
</div>
CSS Styles
.login-container {
min-height: 100vh;
background: url('https://cdn.pixabay.com/photo/2023/10/23/13/20/abstract-8336084_1280.png') no-repeat center center fixed;
background-size: cover;
display: flex;
align-items: center;
justify-content: center;
}
.login-form {
background-color: rgba(255, 255, 255, 0.8);
padding: 40px;
border-radius: 5px;
width: 100%;
max-width: 400px;
}
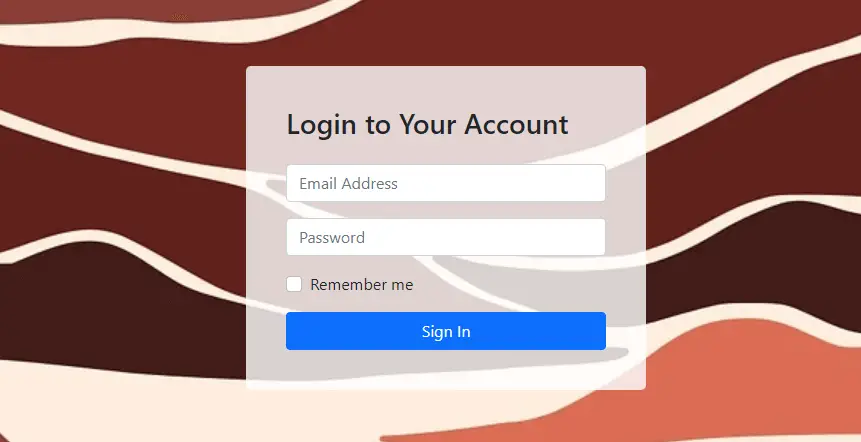
Bootstrap 5 Side Image with Login Form
Create a responsive Bootstrap 5 login form with a side image for an simple design.
<div class="container">
<div class="row align-items-center" style="min-height: 100vh;">
<div class="col-md-6 d-none d-md-block">
<img src="https://cdn.pixabay.com/photo/2023/10/21/21/55/flowers-8332722_640.jpg" alt="Login" class="img-fluid">
</div>
<div class="col-md-6">
<!-- Login Form -->
<h3 class="mb-4 text-center">Please Sign In</h3>
<form>
<div class="mb-3">
<input type="email" class="form-control" placeholder="Email address" required autofocus>
</div>
<div class="mb-3">
<input type="password" class="form-control" placeholder="Password" required>
</div>
<div class="mb-3">
<div class="checkbox">
<label>
<input type="checkbox" value="remember-me"> Remember me
</label>
</div>
</div>
<div class="d-grid">
<button class="btn btn-lg btn-primary btn-block" type="submit">Sign in</button>
</div>
</form>
</div>
</div>
</div>
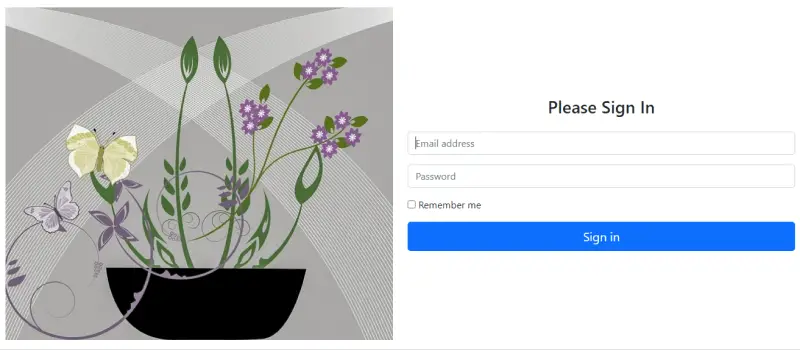
Javed sheikh
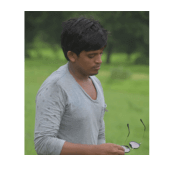
Hello there! I’m Javed Sheikh, a frontend developer with a passion for crafting seamless user experiences. With expertise in JavaScript frameworks like Vue.js, Svelte, and React, I bring creativity and innovation to every project I undertake. From building dynamic web applications to optimizing user interfaces,