In this tutorial, I’ll guide you through the process of installing Ant Design in a Vue 3 project with TypeScript using the Vite build tool.
Create Vue 3 Project
Install a Vue 3 project with the following command in your terminal:
# With NPM:
npm create vite@latest
# With Yarn:
yarn create vite
# With PNPM:
pnpm create vite
Next, give project name and select vue project.
$ npm create vite@latest
√ Project name: ... ant-vue
? Select a framework: » - Use arrow-keys. Return to submit.
Vanilla
> Vue
React
Preact
Lit
Svelte
Others
Now select Customize with create-vue .
√ Project name: ... ant-vue
√ Select a framework: » Vue
? Select a variant: » - Use arrow-keys. Return to submit.
JavaScript
TypeScript
> Customize with create-vue ↗
Nuxt ↗
Select your Vue 3 project requirements, such as TypeScript and routes.
√ Add TypeScript? ... No / Yes
√ Add JSX Support? ... No / Yes
√ Add Vue Router for Single Page Application development? ... No / Yes
√ Add Pinia for state management? ... No / Yes
√ Add Vitest for Unit Testing? ... No / Yes
√ Add an End-to-End Testing Solution? » No
√ Add ESLint for code quality? ... No / Yes
Move to project dir.
cd ant-vue
npm install
npm run dev
Install Ant Design In Vue 3
Run the command below to install Ant Design in Vue 3.
# With NPM:
npm install ant-design-vue --save
# With Yarn:
yarn add ant-design-vue
Next, manually import Ant Design stylesheets in main.ts
.
import 'ant-design-vue/dist/antd.css'
Use Ant Design global registration for all components.
main.ts
import { createApp } from 'vue';
import Antd from 'ant-design-vue';
import App from './App';
import 'ant-design-vue/dist/antd.css';
const app = createApp(App);
app.use(Antd).mount('#app');
Test the Vue 3 Ant Design button component.
HomeView.vue
<script setup lang="ts">
import TheWelcome from '../components/TheWelcome.vue'
</script>
<template>
<main>
<TheWelcome />
<a-button type="primary">Primary Button</a-button>
<a-button>Default Button</a-button>
<a-button type="dashed">Dashed Button</a-button>
<a-button type="text">Text Button</a-button>
<a-button type="link">Link Button</a-button>
</main>
</template>
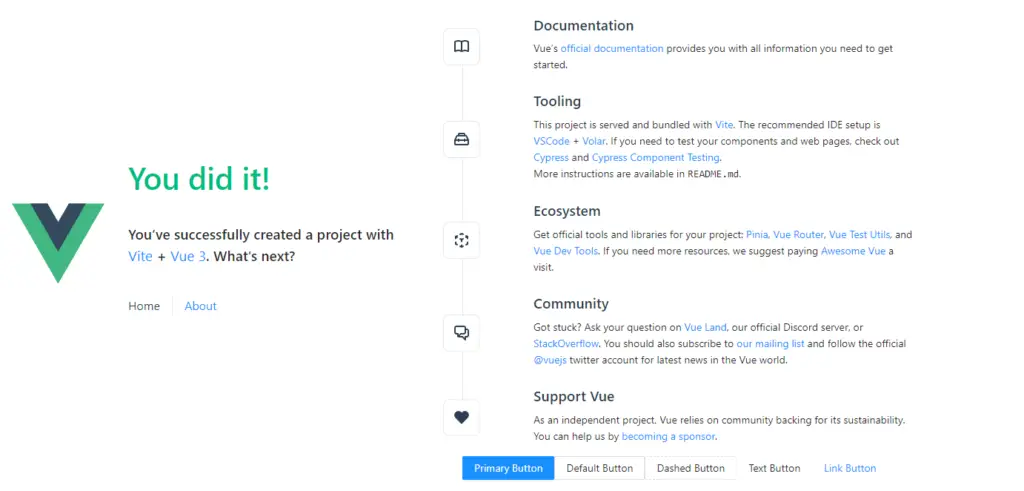
Javed sheikh
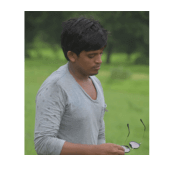
Hello there! I’m Javed Sheikh, a frontend developer with a passion for crafting seamless user experiences. With expertise in JavaScript frameworks like Vue.js, Svelte, and React, I bring creativity and innovation to every project I undertake. From building dynamic web applications to optimizing user interfaces,