In this tutorial, you will learn how to create outlined buttons using HTML and CSS. We will also learn to create Dashed Outline Buttons, Double Outline Buttons, and Gradient Outline Buttons.
Starting HTML Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Outline Buttons</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<button class="outline-btn">Button 1</button>
<button class="outline-btn">Button 2</button>
<button class="outline-btn">Button 3</button>
</body>
</html>
CSS Styles
.outline-btn {
background-color: transparent;
border: 2px solid #007BFF;
color: #007BFF;
padding: 10px 20px;
font-size: 16px;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s, color 0.3s;
}
.outline-btn:hover {
background-color: #007BFF;
color: #ffffff;
}
.outline-btn:active {
background-color: #0056b3;
}
.outline-btn:focus {
outline: none;
box-shadow: 0 0 0 3px rgba(0, 123, 255, 0.5);
}

Dashed Outline Button
This design uses a dashed border around the button for a distinctive look.
<button class="dashed-btn">Dashed Button</button>
CSS Styles
.dashed-btn {
background-color: transparent;
border: 2px dashed #FF5733;
color: #FF5733;
padding: 10px 20px;
font-size: 16px;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s, color 0.3s;
}
.dashed-btn:hover {
background-color: #FF5733;
color: #ffffff;
}
The main difference here is using the dashed value for the border property to create a dashed outline.

Double Outline Button
This design has two borders, giving the button a grooved appearance.
<button class="double-outline-btn">Double Outline Button</button>
CSS Styles
.double-outline-btn {
background-color: transparent;
border: solid 2px #33FF57;
padding: 10px 20px;
position: relative;
color: #33FF57;
font-size: 16px;
cursor: pointer;
transition: background-color 0.3s, color 0.3s;
}
.double-outline-btn:before {
content: '';
position: absolute;
top: -5px;
right: -5px;
bottom: -5px;
left: -5px;
border: solid 2px #33FF57;
pointer-events: none;
}
.double-outline-btn:hover {
background-color: #33FF57;
color: #ffffff;
}
We utilize the :before pseudo-element to achieve the double border. By positioning this pseudo-element around the button and giving it a border, it appears as if the button has two borders.

Gradient Outline Button
This button showcases a gradient as its border.
<button class="gradient-outline-btn">Gradient Outline Button</button>
CSS Styles
.gradient-outline-btn {
background-color: transparent;
border: 2px solid;
border-image: linear-gradient(45deg, #FFC300, #FF5733);
border-image-slice: 1;
color: #FF5733;
padding: 10px 20px;
font-size: 16px;
border-radius: 5px;
cursor: pointer;
transition: color 0.3s;
}
.gradient-outline-btn:hover {
color: #FFC300;
}
We use the border-image property to set a gradient for the button’s border. The border-image-slice is necessary for the gradient border to display correctly.

Jameel Rathore
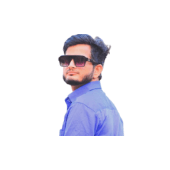
Hello! My name is Jameel Rathore, and I’m excited to share insights on web development topics. With expertise in HTML, CSS, and WordPress, I blend creativity with technical know-how in every article. My goal is to offer valuable insights and tips,