In this tutorial, learn to create a dropdown menu in Nuxt 3 using Tailwind CSS. Discover functionalities such as dropdowns with icons and menu activation on click and hover. Ensure Tailwind CSS is installed and configured in your Nuxt 3 project before starting.
Install Tailwind CSS in Nuxt 3 with NuxtTailwind Module
NuxtJS 3 with Tailwind CSS Dropdown Menu on Click
Vue
<script setup>
import { ref } from 'vue'
const isDropdownOpen = ref(false)
const toggleDropdown = () => {
isDropdownOpen.value = !isDropdownOpen.value
}
</script>
<template>
<div class="relative inline-block text-left">
<button @click="toggleDropdown"
class="inline-flex justify-center w-full px-4 py-2 text-sm font-medium text-gray-700 bg-white border border-gray-300 rounded-md shadow-sm hover:bg-gray-50 focus:outline-none">
Dropdown
</button>
<div v-if="isDropdownOpen"
class="absolute z-10 w-56 mt-2 origin-top-right bg-white rounded-md shadow-lg left-0 ring-1 ring-black ring-opacity-5 focus:outline-none">
<div class="py-1">
<!-- Dropdown links or content here -->
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100">Link 1</a>
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100">Link 2</a>
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100">Link 3</a>
</div>
</div>
</div>
</template>
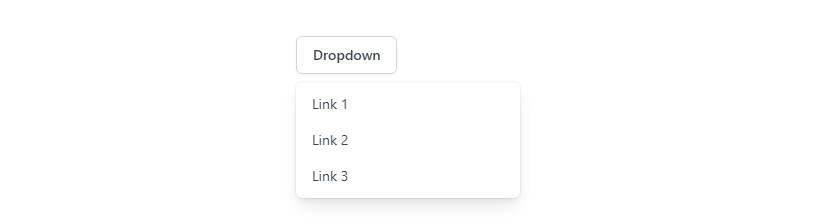
Create a dropdown menu in Nuxt 3 using TypeScript.
Vue
<script setup lang="ts">
import { ref, defineProps } from 'vue';
const props = defineProps(['/* List of your props here */']);
const isDropdownOpen = ref(false);
const toggleDropdown = (): void => {
isDropdownOpen.value = !isDropdownOpen.value;
};
</script>
NuxtJS 3 with Tailwind CSS Dropdown Menu with SVG Icon
Vue
<script setup>
import { ref } from 'vue';
const isDropdownOpen = ref(false);
const toggleDropdown = () => {
isDropdownOpen.value = !isDropdownOpen.value;
};
</script>
<template>
<div class="relative">
<div class="inline-block text-left">
<button @click="toggleDropdown"
class="inline-flex items-center px-4 py-2 text-sm font-medium text-gray-700 bg-white border border-gray-300 rounded-md shadow-sm hover:bg-gray-50 focus:outline-none">
Dropdown button with Icon
<svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" stroke-width="1.5" stroke="currentColor"
class="w-5 h-5 ml-2">
<path stroke-linecap="round" stroke-linejoin="round" d="M19.5 8.25l-7.5 7.5-7.5-7.5" />
</svg>
</button>
<div v-if="isDropdownOpen"
class="absolute z-10 w-56 mt-2 bg-white rounded-md shadow-lg ring-1 ring-black ring-opacity-5 focus:outline-none">
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100">Dashboard</a>
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100">Settings</a>
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100">Earnings</a>
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100">Sign out</a>
</div>
</div>
</div>
</template>
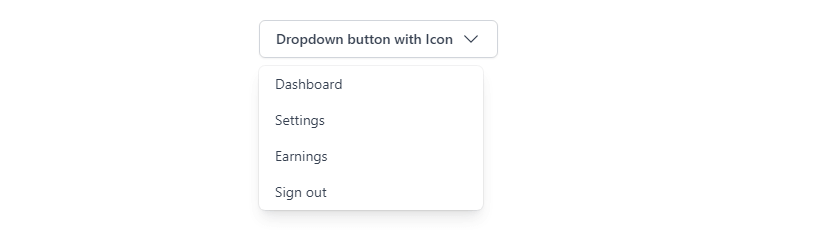
NuxtJS 3 with Tailwind CSS Dropdown Menu on Hover
Vue
<script setup>
import { ref } from 'vue';
const isDropdownOpen = ref(false);
const onMouseLeave = (event) => {
// Check if the mouse is leaving both the trigger and the dropdown
if (!event.relatedTarget || !event.currentTarget.contains(event.relatedTarget)) {
isDropdownOpen.value = false;
}
};
</script>
<template>
<div class="relative inline-block text-left" @mouseleave="onMouseLeave">
<!-- Trigger -->
<div @mouseenter="isDropdownOpen = true">
<button
class="inline-flex items-center px-4 py-2 text-sm font-medium text-gray-700 bg-white border border-gray-300 rounded-md shadow-sm hover:bg-gray-50 focus:outline-none">
Dropdown Hover
<svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" stroke-width="1.5" stroke="currentColor"
class="w-5 h-5 mr-2">
<path stroke-linecap="round" stroke-linejoin="round" d="M19.5 8.25l-7.5 7.5-7.5-7.5" />
</svg>
</button>
</div>
<!-- Dropdown -->
<div v-show="isDropdownOpen"
class="absolute z-10 w-56 mt-2 bg-white rounded-md shadow-lg ring-1 ring-black ring-opacity-5"
@mouseleave="onMouseLeave">
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100">Dashboard</a>
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100">Settings</a>
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100">Earnings</a>
<a href="#" class="block px-4 py-2 text-sm text-gray-700 hover:bg-gray-100">Sign out</a>
</div>
</div>
</template>
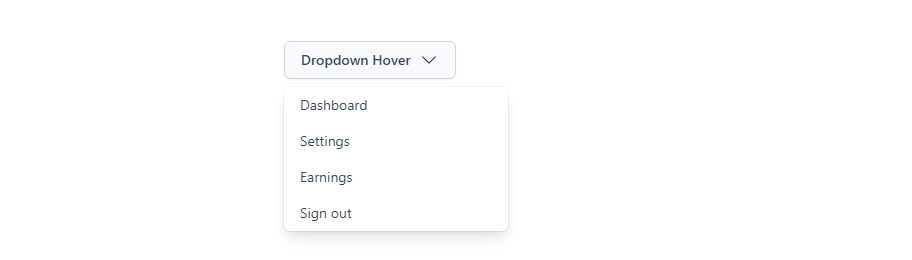
Javed sheikh
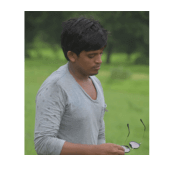
Hello there! I’m Javed Sheikh, a frontend developer with a passion for crafting seamless user experiences. With expertise in JavaScript frameworks like Vue.js, Svelte, and React, I bring creativity and innovation to every project I undertake. From building dynamic web applications to optimizing user interfaces,