In this tutorial, you will learn how to create Real Estate Card Design using HTML and CSS. We will also learn to create Real Estate Card with a Rating, Real Estate Responsive Card with a Footer Section.
Starting HTML Structure
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Real Estate Card</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<div class="card">
<img
src="https://cdn.pixabay.com/photo/2014/07/10/17/18/large-home-389271_1280.jpg"
alt="House"
class="card-img"
/>
<div class="card-content">
<h2 class="card-title">Luxury Villa</h2>
<p class="card-info">123 Main St, Some City</p>
<p class="card-info">$1,200,000</p>
<button class="card-btn">View Details</button>
</div>
</div>
</body>
</html>
CSS Styles
CSS
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f4f4f4;
}
.card {
width: 300px;
border-radius: 8px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
overflow: hidden;
background-color: #ffffff;
margin: 16px;
}
.card-img {
width: 100%;
height: 200px;
object-fit: cover;
}
.card-content {
padding: 16px;
}
.card-title {
font-size: 1.5em;
margin-bottom: 8px;
}
.card-info {
font-size: 1em;
color: #666;
margin-bottom: 8px;
}
.card-btn {
display: inline-block;
padding: 10px 20px;
background-color: #007bff;
color: #fff;
text-decoration: none;
border: none;
border-radius: 4px;
cursor: pointer;
}
.card-btn:hover {
background-color: #0056b3;
}
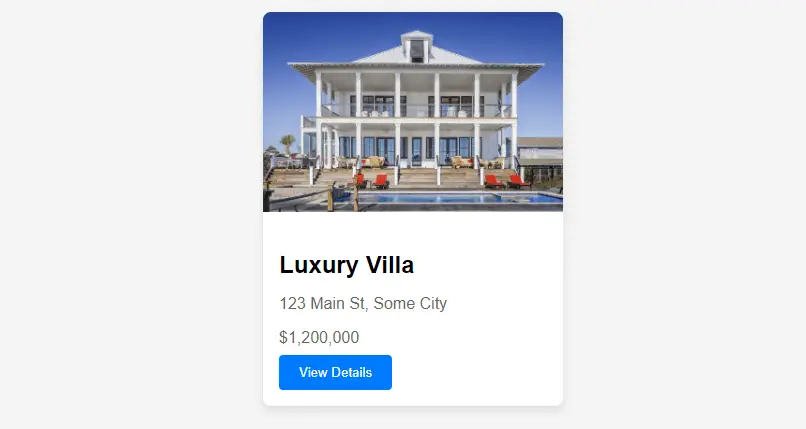
Real Estate Card with a Rating
This example includes a star rating system to indicate the property’s reviews.
HTML
<div class="card-container">
<div class="card-rating">
<img
src="https://cdn.pixabay.com/photo/2020/06/25/10/21/architecture-5339245_1280.jpg"
alt="House"
class="card-img"
/>
<div class="card-content">
<h2 class="card-title">Modern Apartment</h2>
<p class="card-address">789 Boulevard St, New City</p>
<p class="card-price">$1,500 / month</p>
<div class="card-stars">★★★★☆</div>
<button class="card-btn">Contact Agent</button>
</div>
</div>
</div>
CSS Styles
CSS
body {
font-family: 'Arial', sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.card-container {
width: 400px;
box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2);
transition: 0.3s;
border-radius: 10px;
overflow: hidden;
}
.card-rating {
width: 100%;
display: grid;
grid-template-rows: auto 1fr;
}
.card-img {
width: 100%;
height: auto;
object-fit: cover;
}
.card-content {
padding: 20px;
}
.card-title {
font-size: 24px;
margin-bottom: 8px;
}
.card-address,
.card-price {
color: #333;
margin-bottom: 16px;
}
.card-stars {
color: #ffd700;
font-size: 20px;
margin-bottom: 16px;
}
.card-btn {
background-color: #007bff;
color: white;
border: none;
padding: 10px 20px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
border-radius: 5px;
transition: background-color 0.3s ease;
}
.card-btn:hover {
background-color: #0056b3;
}
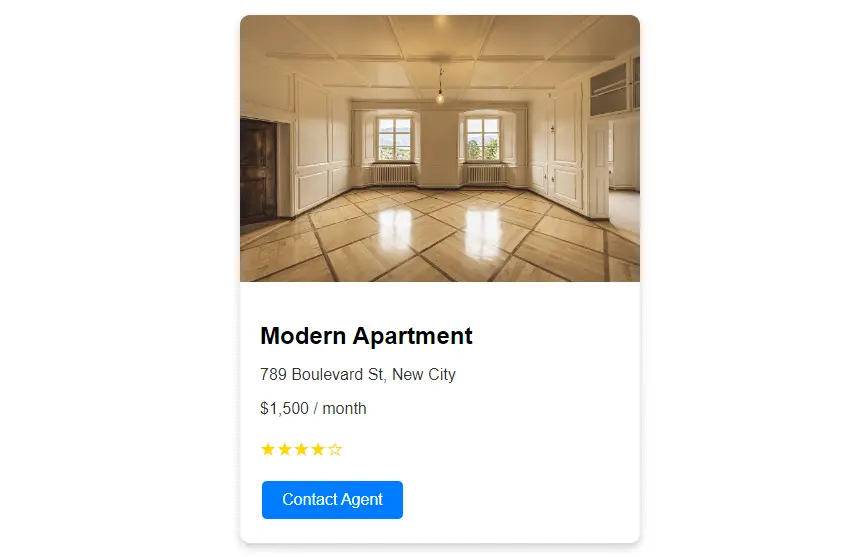
Real Estate Responsive Card with a Footer Section
This example adds a footer section to the card, which could be used to display additional contact information.
HTML
<div class="card-footer">
<img
src="https://cdn.pixabay.com/photo/2017/04/13/17/48/floor-2228277_640.jpg"
alt="House"
class="card-img"
/>
<div class="card-content">
<h2 class="card-title">Beachfront Villa</h2>
<p class="card-info">101 Coastal Rd, Beach City</p>
<p class="card-info">$3,200,000</p>
<button class="card-btn">Explore More</button>
</div>
<div class="card-footer-content">
<a href="#" class="footer-link">More Listings</a>
<a href="#" class="footer-link">Contact Us</a>
</div>
</div>
CSS Styles
CSS
body {
font-family: 'Arial', sans-serif;
margin: 0;
padding: 20px;
background-color: #f7f7f7;
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
}
.card-footer {
width: 100%;
max-width: 400px;
margin: auto;
overflow: hidden;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2);
background: #ffffff;
display: flex;
flex-direction: column;
}
.card-img {
width: 100%;
height: auto;
display: block;
}
.card-content {
padding: 20px;
}
.card-title {
font-size: 24px;
margin-bottom: 8px;
}
.card-info {
color: #333;
margin-bottom: 16px;
}
.card-btn {
background-color: #007bff;
color: white;
border: none;
padding: 10px 20px;
text-align: center;
text-decoration: none;
font-size: 16px;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
}
.card-btn:hover {
background-color: #0056b3;
}
.card-footer-content {
background: #f8f9fa;
padding: 10px 20px;
display: flex;
justify-content: space-between;
align-items: center;
}
.footer-link {
text-decoration: none;
color: #007bff;
font-weight: bold;
transition: color 0.3s ease;
}
.footer-link:hover {
color: #0056b3;
}
@media (max-width: 768px) {
.card-footer {
margin: 20px;
}
.card-content {
padding: 15px;
}
.card-title {
font-size: 20px;
}
.card-footer-content {
flex-direction: column;
align-items: stretch;
}
.footer-link {
margin-bottom: 10px;
}
}
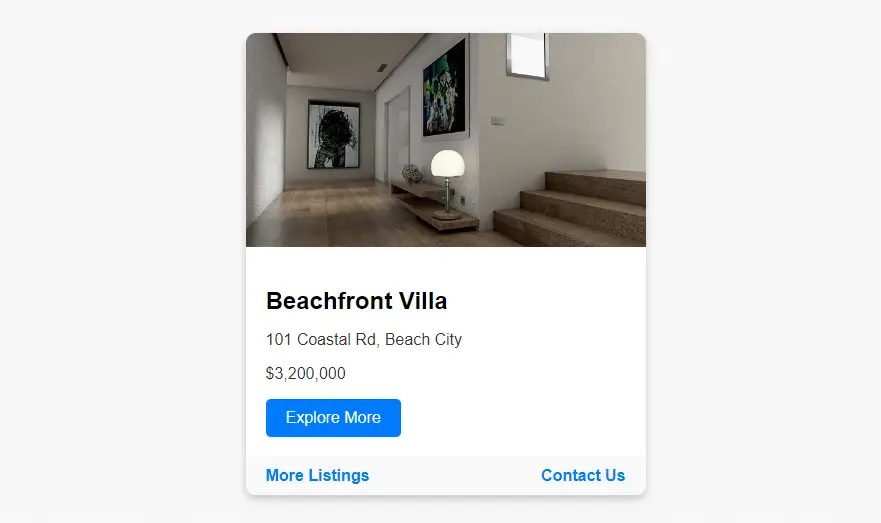
Jameel Rathore
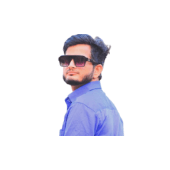
Hello! My name is Jameel Rathore, and I’m excited to share insights on web development topics. With expertise in HTML, CSS, and WordPress, I blend creativity with technical know-how in every article. My goal is to offer valuable insights and tips,