In this tutorial, we will explore how to use Vuetify.js 3 in Vue.js 3 to create Carousel components. We’ll cover features like arrow navigation and automatic slide transitions. Before we begin, ensure that you have installed and configured Vuetify 3 in Vue 3.
How to Install Vuetify 3 in Vue 3
Vuetify 3 Carousel Slider Examples
1. Vuetify 3 Create a simple carousel using the v-carousel
and v-carousel-item
components.
<template>
<v-carousel>
<v-carousel-item src="https://cdn.vuetifyjs.com/images/cards/docks.jpg" cover></v-carousel-item>
<v-carousel-item src="https://cdn.vuetifyjs.com/images/cards/hotel.jpg" cover></v-carousel-item>
<v-carousel-item src="https://cdn.vuetifyjs.com/images/cards/sunshine.jpg" cover></v-carousel-item>
</v-carousel>
</template>
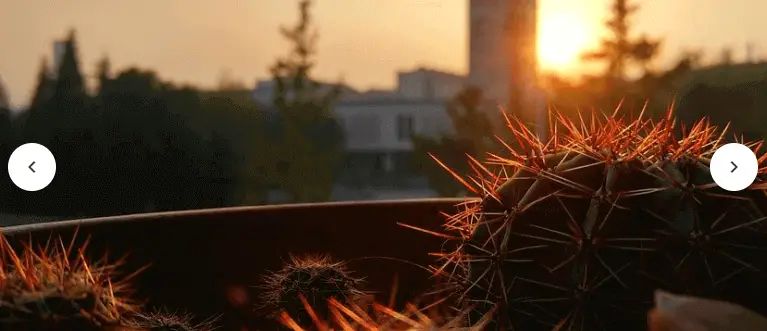
2. Vuetify 3 Vue 3 carousel Show arrows on hover using show-arrows="hover"
.
<template>
<v-carousel show-arrows="hover">
<v-carousel-item src="https://cdn.vuetifyjs.com/images/cards/docks.jpg" cover></v-carousel-item>
<v-carousel-item src="https://cdn.vuetifyjs.com/images/cards/hotel.jpg" cover></v-carousel-item>
<v-carousel-item src="https://cdn.vuetifyjs.com/images/cards/sunshine.jpg" cover></v-carousel-item>
</v-carousel>
</template>
3. Vuetify 3 Vue 3 carousel card slider with delimiter.
<template>
<v-card elevation="24" max-width="444" class="mx-auto">
<v-carousel :continuous="false" :show-arrows="false" hide-delimiter-background delimiter-icon="mdi-square"
height="300">
<v-carousel-item v-for="(slide, i) in slides" :key="i">
<v-sheet :color="colors[i]" height="100%" tile>
<div class="d-flex fill-height justify-center align-center">
<div class="text-h2">
{{ slide }} Slide
</div>
</div>
</v-sheet>
</v-carousel-item>
</v-carousel>
</v-card>
</template>
<script>
export default {
data() {
return {
colors: [
'green',
'secondary',
'yellow darken-4',
'red lighten-2',
'orange darken-1',
],
slides: [
'First',
'Second',
'Third',
'Fourth',
'Fifth',
],
}
},
}
</script>
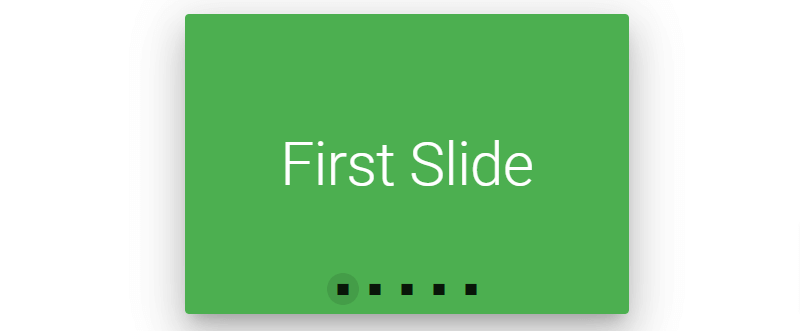
4. Vuetify 3 Vue 3 carousel: Slides automatically transition to the next available every 6 seconds (default).
<template>
<v-carousel cycle height="400" hide-delimiter-background show-arrows="hover">
<v-carousel-item v-for="(slide, i) in slides" :key="i">
<v-sheet :color="colors[i]" height="100%">
<div class="d-flex fill-height justify-center align-center">
<div class="text-h2">
{{ slide }} Slide
</div>
</div>
</v-sheet>
</v-carousel-item>
</v-carousel>
</template>
<script>
export default {
data() {
return {
colors: [
'indigo',
'warning',
'pink darken-2',
'red lighten-1',
'deep-purple accent-4',
],
slides: [
'First',
'Second',
'Third',
'Fourth',
'Fifth',
],
}
},
}
</script>
5. Vuetify 3 Vue 3 carousel slides with Previous slide button and Next slide button.
<template>
<v-carousel height="400" show-arrows hide-delimiter-background>
<template v-slot:prev="{ props }">
<v-btn variant="elevated" color="success" @click="props.onClick">Previous slide</v-btn>
</template>
<template v-slot:next="{ props }">
<v-btn variant="elevated" color="info" @click="props.onClick">Next slide</v-btn>
</template>
<v-carousel-item v-for="(slide, i) in slides" :key="i">
<v-sheet :color="colors[i]" height="100%">
<div class="d-flex fill-height justify-center align-center">
<div class="text-h2">
{{ slide }} Slide
</div>
</div>
</v-sheet>
</v-carousel-item>
</v-carousel>
</template>
<script>
export default {
data() {
return {
colors: [
'indigo',
'warning',
'pink darken-2',
'red lighten-1',
'deep-purple accent-4',
],
slides: [
'First',
'Second',
'Third',
'Fourth',
'Fifth',
],
}
},
}
</script>
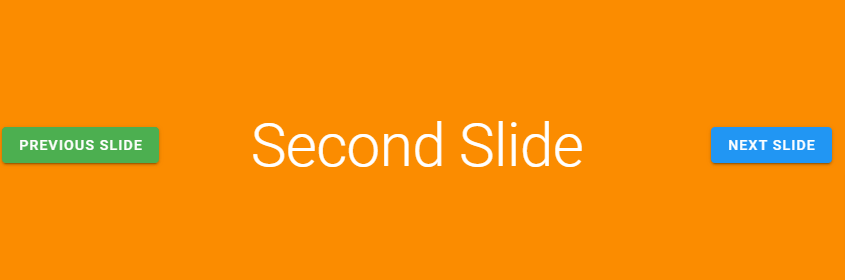
6. Vuetify 3 Vue 3 carousel slides show a linear progress bar with the progress prop.
<template>
<v-carousel height="400" hide-delimiters progress="primary">
<v-carousel-item v-for="(slide, i) in slides" :key="i">
<v-sheet height="100%">
<div class="d-flex fill-height justify-center align-center">
<div class="text-h2">
{{ slide }} Slide
</div>
</div>
</v-sheet>
</v-carousel-item>
</v-carousel>
</template>
<script>
export default {
data() {
return {
slides: [
'First',
'Second',
'Third',
'Fourth',
'Fifth',
],
}
},
}
</script>
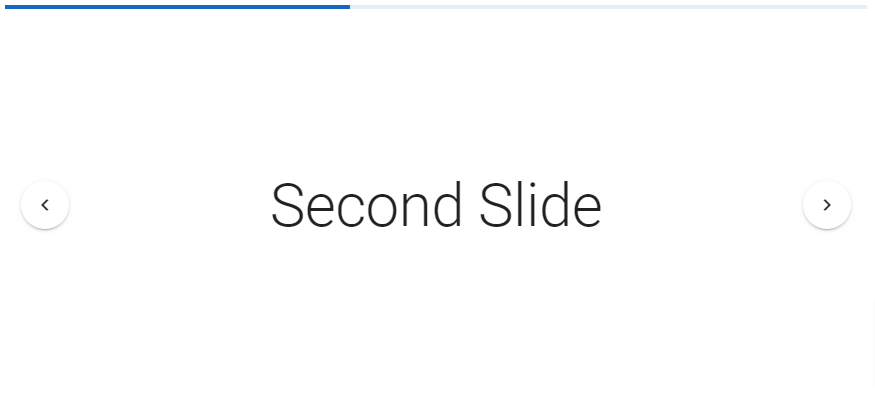
Javed sheikh
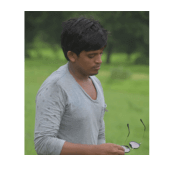
Hello there! I’m Javed Sheikh, a frontend developer with a passion for crafting seamless user experiences. With expertise in JavaScript frameworks like Vue.js, Svelte, and React, I bring creativity and innovation to every project I undertake. From building dynamic web applications to optimizing user interfaces,